A key-value store is a simple yet powerful type of database that allows you to associate unique keys with corresponding values.
While it is conceptually similar to a Python dictionary, a key-value store can be enhanced to support more advanced features like persistence, expiration, and encryption.
In this article, we’ll walk through how to create a key-value store in Python, starting with an in-memory version and progressing to more advanced implementations.
By the end, you'll have the tools and knowledge to create your own key-value store tailored to your application's specific needs.
What is a Key-Value Store?
A key-value store allows you to map keys to specific values, providing fast lookups, inserts, and updates.
These systems are used in applications like caching, configuration storage, and even large distributed databases like Redis or DynamoDB. NoSQL databases often use key-value stores as one of their underlying data models.
Unlike relational databases, key-value stores do not have structured tables, making them faster and more lightweight in specific use cases.
Prerequisites
To follow along this article, you’ll need:
- Basic Python knowledge: Familiarity with dictionaries, file handling, and classes.
- Python 3.x installed: Make sure Python is installed on your system.
- Optional libraries: For encryption, you’ll need the
cryptography
library. For storage optimization, theshelve
module is part of Python’s standard library.
Create an In-Memory Key-Value Store
An in-memory key-value store exists only while the program is running, and all data is lost when the program stops.
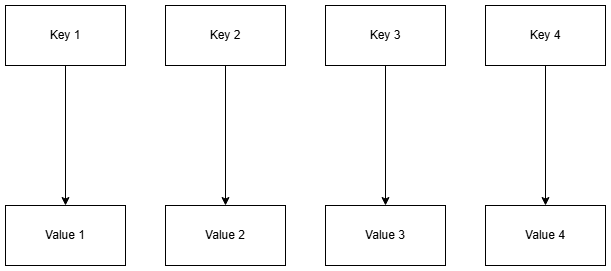
This is the simplest form of a key-value store and serves as a strong foundation for more advanced implementations.
class InMemoryKeyValueStore:
def __init__(self):
"""Initialize an empty dictionary to store key-value pairs."""
self.store = {}
def set(self, key, value):
"""Store a key-value pair."""
self.store[key] = value
def get(self, key):
"""Retrieve the value for a given key."""
return self.store.get(key)
def delete(self, key):
"""Remove a key-value pair."""
if key in self.store:
del self.store[key]
def keys(self):
"""Return a list of all keys."""
return list(self.store.keys())
if __name__ == '__main__':
# Usage example
store = InMemoryKeyValueStore()
store.set('name', 'Alice')
print(store.get('name')) # Output: Alice
store.delete('name')
print(store.get('name')) # Output: None
This code defines a simple in-memory key-value store implemented as a Python class named InMemoryKeyValueStore
.
The class initializes an empty dictionary to store key-value pairs and provides methods to interact with this store:
- The
__init__
method sets up the dictionary. - The
set
method allows adding or updating key-value pairs. - The
get
method retrieves values associated with specific keys, returningNone
if the key does not exist. - The
delete
method removes key-value pairs from the store if the key is present. - The
keys
method returns a list of all keys currently stored in the dictionary.
The usage example demonstrates how to create an instance of the InMemoryKeyValueStore
class, set a key-value pair, retrieve the value, delete the key, and attempt to retrieve the value again after deletion.
This simple approach is useful for applications that need a temporary, fast key-value store with minimal overhead.
Create a File-Based Key-Value Store
To make the store persistent, we’ll save key-value pairs to a file. We’ll use the json
module to serialize and deserialize data.
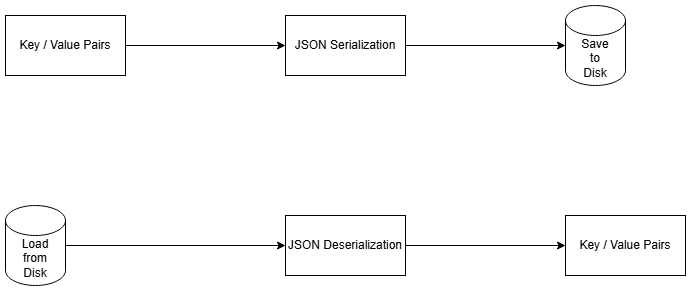
This approach allows you to store data across program runs, but it has some limitations in terms of speed and scalability for large datasets.
This article is for subscribers only
To continue reading this article, just register your email and we will send you access.
Subscribe NowAlready have an account? Sign In