As technology evolves, developers are increasingly looking to bring the flexibility and power of web applications to the desktop.
Electron.js is a popular framework that allows developers to create cross-platform desktop applications using JavaScript, HTML, and CSS—making it an ideal choice for web developers seeking to create desktop apps without learning new languages or technologies.
In this guide, we’ll walk through the steps of building a simple desktop app using Electron, including how to set up your environment, create a basic app, add a feature to fetch quotes from an API and package your app for deployment.
What is Electron?
Electron is an open-source framework developed by GitHub that lets developers build desktop apps using familiar web technologies.
Electron packages web applications with Node.js and Chromium, enabling them to run as standalone applications on Windows, macOS, and Linux.
This blend of web and native application capabilities has allowed popular applications like Slack, Visual Studio Code, and Discord to be built with Electron.
One of the standout features of Electron for me is how it combines the two worlds: web technologies for UI and Node.js for backend functionalities.
This can make transitioning to desktop app development smoother for web developers, though it comes with some trade-offs, like app size and memory usage, which we’ll discuss later.
Why Use Electron?
Although I was initially drawn to Electron for its cross-platform support, I quickly realized its potential as a one-stop solution for creating complex applications without the hassle of maintaining separate native codebases.
However, keep in mind that Electron apps can be relatively large in size, especially when compared to truly native apps, due to bundling Chromium and Node.js.
Key Benefits:
- Cross-platform Compatibility: Electron lets you write code once and deploy it on multiple operating systems, making it perfect for reaching a broad audience without needing to manage separate codebases.
- Use of Web Technologies: Electron allows you to create rich, interactive UIs with HTML, CSS, and JavaScript—perfect for developers who are already comfortable in the web development ecosystem.
- Node.js Integration: Electron supports the use of Node.js modules, enabling developers to add robust backend features, such as file system access, databases, and third-party packages, directly to the desktop app.
Look no further than my latest ebook, "Python Tricks - A Collection of Tips and Techniques".
Get the eBook
Inside, you'll discover a collection of Python secrets that will guide you through a journey of learning how to write cleaner, faster, and more Pythonic code.
Setting Up Your Environment
Before diving into coding, ensure you have Node.js installed. With Node.js, you can use npm (Node Package Manager) to install Electron.
Project Structure: Here’s a minimal project structure for a basic Electron application:
my-electron-app/
├── package.json
├── index.js
└── index.html
Set Up a Project Folder and initialize npm within it to manage dependencies locally:
mkdir my-electron-app && cd my-electron-app
npm init -y
npm install electron --save-dev
Install Electron Globally (for use across projects):
npm install -g electron
Creating a Basic Electron Application
Electron applications rely on two types of processes:
- Main Process: This controls the lifecycle of the application and manages native elements like windows and menus.
- Renderer Process: This handles the UI of each window, which is essentially a web page (HTML/CSS/JavaScript) rendered using Chromium.
Step 1: Setting Up the Main Process
In index.js
, create a basic Electron window. This file will act as the entry point to your app.
const { app, BrowserWindow } = require('electron');
function createWindow() {
const win = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
nodeIntegration: true,
contextIsolation: false,
},
});
win.loadFile('index.html');
}
app.whenReady().then(createWindow);
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') app.quit();
});
app.on('activate', () => {
if (BrowserWindow.getAllWindows().length === 0) createWindow();
});
I found the two-process model quite useful.
The main process is like the backbone, responsible for controlling everything behind the scenes, while the renderer handles the visuals.
This separation makes the code more modular and easier to manage, though it can feel a bit tricky initially.
Step 2: Building the Renderer Process
The index.html
file will serve as the user interface for your application.
Here’s a simple example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Electron App</title>
</head>
<body>
<h1>Welcome to Electron!</h1>
<p>This is your first Electron app.</p>
</body>
</html>
Running Your App:
To start your Electron application, add a start script to your package.json
file:
"scripts": {
"start": "electron ."
}
Run your app:
npm start
You should see this application window:
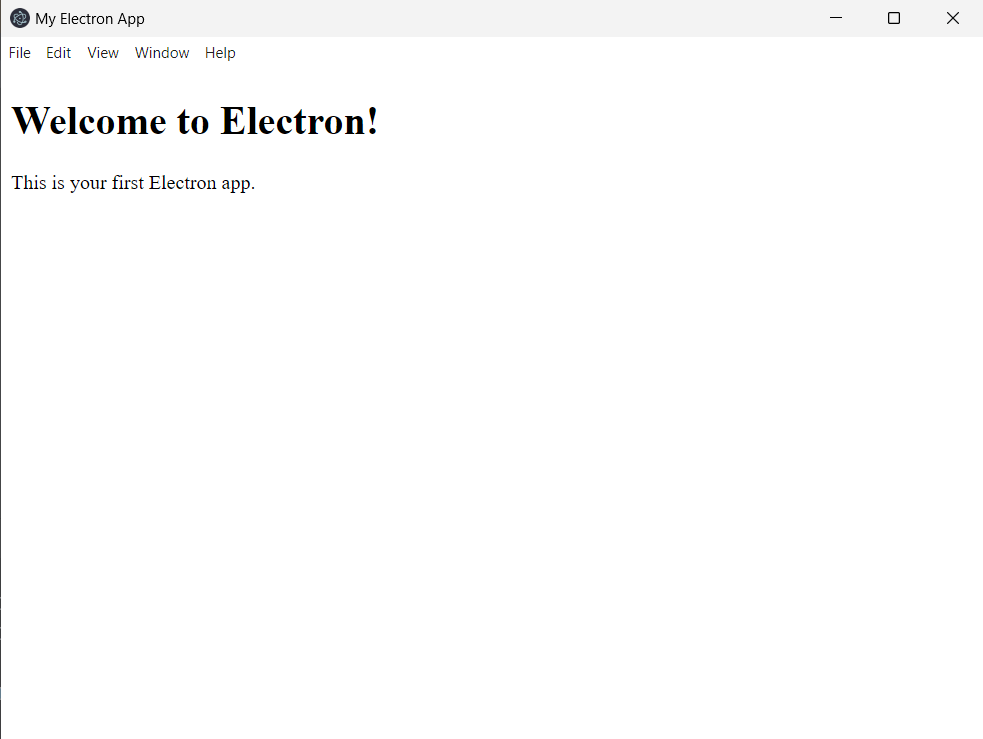
The ability to use HTML and CSS in Electron applications is powerful because it lets you bring responsive web design and even CSS frameworks directly into your app.
However, Electron doesn’t support some web APIs, so always double-check compatibility.
Adding API Integration: Fetching a Random Quote from Quotable API
Let’s add some interactivity by fetching and displaying a random quote from Quotable, a free API for random quotes.
Step 1: Update HTML for Interactivity
Add a button to fetch a quote and a placeholder for the quote in index.html
.
<body>
<h1>Random Quote App</h1>
<button id="fetch-quote">Get a Quote</button>
<p id="quote">Press the button to get a quote...</p>
<script src="renderer.js"></script>
</body>
Step 2: Create the Renderer Script (renderer.js
)
In renderer.js
, add JavaScript to fetch and display the quote.
This article is for paid members only
To continue reading this article, upgrade your account to get full access.
Subscribe NowAlready have an account? Sign In