API testing tools like Postman are essential for developers working with APIs.
However, creating a custom tool tailored to specific needs can offer more control and better integration with your projects.
In this article, we'll guide you through building your own basic Postman-like application in Python using the ttkbootstrap library, which provides a modern and visually appealing interface.
What is ttkbootstrap?
ttkbootstrap is a library that enhances the traditional ttk module in tkinter by adding modern themes and additional widgets.
It allows developers to create beautiful and responsive graphical user interfaces (GUIs) with ease.
Building the Postman-like Application
We will create a simple GUI application that allows users to enter an API endpoint, select the request method (GET or POST), add headers and a request body, include authentication (basic and API token), and display the response.
We'll build this application step-by-step, incrementally adding different functionalities.
Get "Python's Magic Methods - Beyond __init__ and __str__"
Magic methods are not just syntactic sugar, they're powerful tools that can significantly improve the functionality and performance of your code. With this book, you'll learn how to use these tools correctly and unlock the full potential of Python.
Step 1: Basic Postman
The application will have the following features:
- A text entry field for the URL.
- A dropdown menu to select the HTTP method (GET, POST, PUT, DELETE).
- A button to send the HTTP request.
- A text area to display the response from the server.
Setting Up the Environment
Before diving into the code, ensure you have the necessary libraries installed. You can install requests
and ttkbootstrap
using pip:
pip install requests ttkbootstrap
The Code
Here is the code for our basic Postman application:
import requests
from tkinter import StringVar, Text, messagebox
import ttkbootstrap as ttk
class PostmanApp(ttk.Window):
def __init__(self):
super().__init__(themename="darkly")
self.title("Basic Postman")
self.geometry("800x600")
# URL Entry
self.url_label = ttk.Label(self, text="URL:")
self.url_label.grid(row=0, column=0, padx=10, pady=10)
self.url_entry = ttk.Entry(self, width=50)
self.url_entry.grid(row=0, column=1, padx=10, pady=10)
# Method Dropdown
self.method_label = ttk.Label(self, text="Method:")
self.method_label.grid(row=1, column=0, padx=10, pady=10)
self.method_var = StringVar(value="GET")
self.method_dropdown = ttk.Combobox(self, textvariable=self.method_var, values=["GET", "POST", "PUT", "DELETE"])
self.method_dropdown.grid(row=1, column=1, padx=10, pady=10)
# Send Button
self.send_button = ttk.Button(self, text="Send", command=self.send_request)
self.send_button.grid(row=2, column=1, padx=10, pady=10)
# Response Text
self.response_text = Text(self, height=20, width=95)
self.response_text.grid(row=3, column=0, columnspan=3, padx=10, pady=10)
def send_request(self):
url = self.url_entry.get()
method = self.method_var.get()
try:
if method == "GET":
response = requests.get(url)
elif method == "POST":
response = requests.post(url)
elif method == "PUT":
response = requests.put(url)
elif method == "DELETE":
response = requests.delete(url)
else:
messagebox.showerror("Error", "Invalid HTTP method.")
return
self.response_text.delete(1.0, ttk.END)
self.response_text.insert(ttk.END, response.text)
except Exception as e:
messagebox.showerror("Error", str(e))
if __name__ == "__main__":
app = PostmanApp()
app.mainloop()
Running the Application
To run the application, execute the Python script. The GUI will appear, allowing you to enter a URL, select an HTTP method, and view the response:
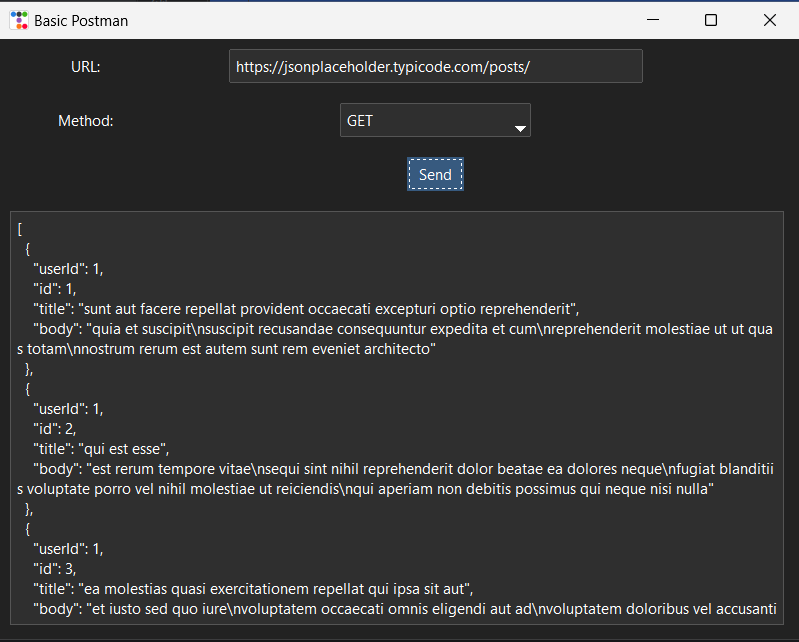
Step 2: Adding Headers and Body
This version includes additional functionality for setting headers and body in the requests:
- Text entry fields for headers and body, allowing JSON input.
The Code
Below is the code changes for our enhanced Postman application:
This article is for paid members only
To continue reading this article, upgrade your account to get full access.
Subscribe NowAlready have an account? Sign In