In this tutorial, I will walk you through the process of creating a simple yet engaging Pong game using the Pygame library in Python.
By the end of this tutorial, you will have a fully functional Pong game that you can play and customize to your liking:
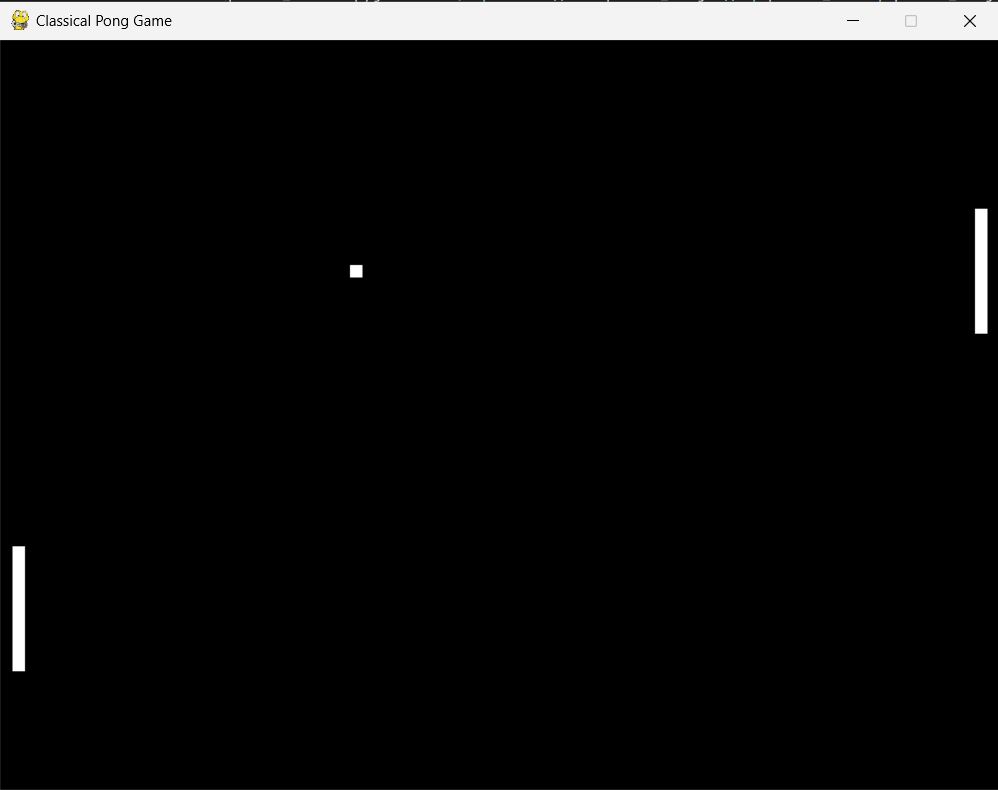
Check out also the YouTube video:
Creating a Classical Pong Game with PyGame
Prerequisites
Before we begin, make sure you have the following prerequisites installed:
- Python 3.x: You can download the latest version of Python from the official website: https://www.python.org/downloads/
- Pygame: Once you have Python installed, you can install Pygame using
pip
by running the following command:pip install pygame
Step 1: Initializing Pygame and Setting up Constants
First, let's import the necessary libraries and initialize Pygame:
import random
import pygame
import sys
# Initialize Pygame
pygame.init()
Next, we'll set up some constants for our game window, frame rate, and colors:
# Set up some constants
WIDTH, HEIGHT = 800, 600
FPS = 60
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
Step 2: Creating the Game Window
Now, let's create the game window using the dimensions we defined earlier:
# Create the game window
screen = pygame.display.set_mode((WIDTH, HEIGHT))
We'll also set the window title to "Classical Pong Game":
# Set the window title
pygame.display.set_caption("Classical Pong Game")
Step 3: Creating the Paddles
Let's create two paddles, one for the left side and one for the right side of the screen:
# Create the paddles
paddle_width, paddle_height = 10, 100
paddle_left = pygame.Rect(10, HEIGHT//2 - paddle_height//2, paddle_width, paddle_height)
paddle_right = pygame.Rect(WIDTH - paddle_width - 10, HEIGHT//2 - paddle_height//2, paddle_width, paddle_height)
Step 4: Creating the Ball
Next, we'll create the ball that will bounce between the paddles:
# Create the ball
ball_size = 10
ball = pygame.Rect(WIDTH//2 - ball_size//2, HEIGHT//2 - ball_size//2, ball_size, ball_size)
ball_speed = 5
ball_direction = [1, -1] # ball will move to the right and up
Step 5: Setting up the Game Clock
We'll create a game clock to control the frame rate of our game:
# Set up the game clock
clock = pygame.time.Clock()
Step 6: Implementing the Game Loop
Now, let's create the game loop where all the game logic and rendering will take place.
Get the eBook
Inside, you'll discover a plethora of Python secrets that will guide you through a journey of learning how to write cleaner, faster, and more Pythonic code. Whether it's mastering data structures, understanding the nuances of object-oriented programming, or uncovering Python's hidden features, this ebook has something for everyone.
This article is for paid members only
To continue reading this article, upgrade your account to get full access.
Subscribe NowAlready have an account? Sign In