Are you looking to improve your Python skills and become a more efficient and effective developer? Look no further than "Python Tricks - A Collection of Tips and Techniques".
This book is a must-have resource for Python developers of all levels, from beginners just starting out to experienced developers looking to expand their knowledge. It covers a wide range of topics, from basic syntax and data structures to advanced concepts like decorators, generators, and regular expressions.
Each chapter is packed with practical tips and tricks that you can use to improve your Python skills and become more productive in your work. You'll learn how to use list comprehensions, the enumerate and zip functions, and the collections module to work with data more efficiently. You'll discover how to create custom decorators and use class-based decorators to simplify your code. You'll learn how to use regular expressions to extract and manipulate text. And much more!
But "Python Tricks - A Collection of Tips and Techniques" is more than just a collection of tips and tricks. It's a comprehensive guide to mastering Python, with plenty of code examples to help you reinforce your learning. The book is written in a clear and concise style, making it easy to follow along and understand even the most complex concepts.
Whether you're looking to improve your skills for work or for personal projects, "Python Tricks - A Collection of Tips and Techniques" is the perfect resource for you. With this book, you'll be able to take your Python skills to the next level and become a more confident and capable developer.
So what are you waiting for? Get your copy of "Python Tricks - A Collection of Tips and Techniques" today and start mastering Python!
Get a 25% discount with the code PYTHONTRICKS
.
If you have any questions or feedback about the book, please don't hesitate to contact me. I value your input and always looking for ways to improve.
Example Chapter 7 - Regular Expressions Tricks
Regular expressions are a powerful tool for pattern matching and text manipulation in Python. In this chapter, we will cover some tricks for working with regular expressions, including basic regular expressions, regular expression functions in Python, and a code example of extracting email addresses from a string.
Basic Regular Expressions
A regular expression is a pattern that describes a set of strings. Regular expressions are composed of characters and special symbols that have specific meanings.
Here are some basic regular expression patterns:
.
matches any character except a newline- `` matches zero or more occurrences of the previous character
+
matches one or more occurrences of the previous character?
matches zero or one occurrence of the previous character^
matches the beginning of a string$
matches the end of a string[]
matches any character inside the brackets|
matches either the pattern before or after the|
Regular Expression Functions in Python
Python provides several functions in the re
module for working with regular expressions. Here are some of the most commonly used functions:
re.search(pattern, string)
searches for the first occurrence of the pattern in the stringre.findall(pattern, string)
finds all occurrences of the pattern in the string and returns them as a list of stringsre.sub(pattern, replacement, string)
substitutes all occurrences of the pattern in the string with the replacement string
Here is an example of using regular expressions to extract email addresses from a string:
import re
import re
text = "Please send your feedback to john@example.com or jane@example.com"
pattern = r"\\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Z|a-z]{2,}\\b"
emails = re.findall(pattern, text)
print(emails)
# Output
# ['john@example.com', 'jane@example.com']
In this chapter, we have covered some tricks for working with regular expressions in Python. Regular expressions can be a powerful tool for text processing and pattern matching and can help you write more efficient and effective code.
Thank you for reading and I will see you on the Internet.
This post is public so feel free to share it.
If you like my free articles and would want to support my work, consider buying me a coffee:
Are you working on a project thatβs encountering obstacles, or are you envisioning the next groundbreaking web application?
If Python, Django, and AI are the tools you're exploring but you need more in-depth knowledge, you're in the right place!
Get in touch for a 1-hour consultation where I can address your specific challenges.
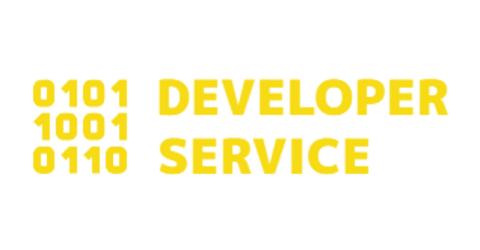